Amazon is one of the most popular and sought-after big tech companies to work in if you’re a software engineer. In this post, we’ll walk you through 75 of the most common questions in technical interviews from Amazon, as well as some tips for landing a job at Amazon as a SWE.
I strongly recommend Tech Interview Pro if you're looking for a proven program that's landed thousands of engineers six-figure jobs at Facebook, Google, Amazon, and Apple, I strongly recommend Tech Interview Pro. With a money-back guarantee and thousands of student success stories, I can't recommend Tech Interview Pro highly enough.
GET TECH INTERVIEW PRO FOR $400 OFF
Offer ends July 28, 2024.Everyone knows that getting a job at one of the big tech companies can be quite challenging.
And in a way, the interviews at companies like Google, Facebook, and Amazon are almost as famous as the companies themselves.
Here are 75 of the most common questions you can expect to see in your Amazon interview: both from a technical and a behavioral perspective.
Amazon Technical Questions
- Write a function that finds the frequency of each element in a given array.
- Given an n-ary tree and some queries for the tree, write a function that prints the preorder traversal of the subtree rooted to a given node.
- Given an array of numbers, write a function that prints the range in which each number is the maximum element.
- Write a function that displays the nodes of a tree in level order.
- Given the entire dictionary of English words, what data structure would you use to efficiently store and match a custom regex string like “D*sk”, where * represents any single character, and return a list of matched words?
- Write a function that counts the occurrence of a number in a given string.
- Given a string of numbers, write a function that places commas in the number so that it becomes easier to read. (Example: 1000000 = 1,000,000)
- Design a system that will keep track of a product and its inventory count.
- Write a function that returns a given integer in string form.
- Given a binary array, write a function that sorts the array in a way that all zeros come to the left and all ones come to the right.
- Design a service that scans photos/videos for any malware.
- Write a method that merges a fixed number of streams containing an infinite sequence of monotonically increasing integers into an output stream of monotonically increasing integers.
- Write a method that returns the value “True” if the number ‘1' appears consecutively throughout the given array.
- Given a list of nodes, with each node having an ID and a parent ID, determine whether the list is given in preorder.
- Given a set of inter-dependent tasks along with the time taken to execute them, write a function that calculates the minimum time required to complete every task.
- Design an authentication system using AWS services like an API gateway and Lambda.
- Design an online chess game.
- Design a movie reviews aggregator system.
- Find the sum of n elements after a kth smallest element in a BST.
- Design a subscription-based sports website that can display scores, game statuses, and the history of any game.
- Given an infinite stream of numbers, write a function that prints the smallest K elements at any point.
- Write a function that finds the minimum length of an unsorted subarray.
- Given an MxN binary matrix, write a method that finds the largest sub-square matrix that only contains 1s.
- Write a function that finds the maximum difference between a node and its ancestor in a binary tree in O(n) time.
- Given an array of integers, write a function that point updates and range sums in minimum time.
- Given a list of numbers of odd length, design an algorithm to decide whether it's possible to remove any number from the list and split the remaining numbers into two sets of equal length with the same sum.
- Given an arithmetic expression, write a method that finds the value of the expression (The only binary operations that are allowed are +, -, *, and /).
- Write an algorithm that finds an unsold item in Amazon's products.
- Given two sorted lists and a number K, create an algorithm that fetches the least K numbers of the two lists.
- Given a dictionary of fixed words, write an algorithm that finds the maximum chain of words that can be formed by any of these words (A chain is formed by picking a word and removing one character from it: this newly formed word should be present in the dictionary).
- Write a function that reconstructs a sentence by adding missing whitespaces.
- Given an array, write a function that finds all valid IP addresses contained within it.
- Given a list of shops (each of which has a list of toys, their prices, and max number of children who can play with it at a time), write a function that outputs a list of best possible toy options from each shop given the number of children who are shopping.
- Given an array of 1 billion numbers with just a few 100 numbers missing, write a function that finds all the missing numbers.
- Design the classes for a Battleship game and write the attack function.
- Write a program that checks whether the data structure given is a valid binary tree or not.
- Design a login system that contains multiple application servers which are login the information to a file system.
- Given two sorted lists and an integer K, write a function that merges the lists up to a maximum of K elements.
- Given a binary tree and a value X, write a method that finds X in the tree and returns its parent.
- Write a function that prints the longest path from root to leaf in a binary tree.
- Write an algorithm for reversing letters of a string in Java.
- Design an ATM machine system.
- Write a function that finds the sum of N elements after the Kth smallest element in a BST.
- Design and implement a traffic control system with pedestrian signal management included.
- Given a binary tree, write a function that prints the nodes in a vertical zig-zag line pattern.
- How would you design a hash table?
- Design a system that will let multiple sellers upload their products to Amazon's seller programs.
- Write a function that determines if the given doubly linked list is a palindrome or not.
- Given a sorted array that has been rotated N number of times, write a function that finds the value of N.
- Given a binary tree and a target number, return whether or not there exists a path that can create the target number.
Amazon Technical Answer Examples
All answers written in C++.
Coding Example #1: Given an infinite stream of numbers, write a function that prints the smallest K elements at any point
#include <climits>
#include <iostream>
using namespace std;
// Prototype of a utility function
// to swap two integers
void swap(int* x, int* y);
// A class for Min Heap
class MinHeap {
int* harr; // pointer to array of elements in heap
int capacity; // maximum possible size of min heap
int heap_size; // Current number of elements in min heap
public:
MinHeap(int a[], int size); // Constructor
void buildHeap();
void MinHeapify(
int i); // To minheapify subtree rooted with index i
int parent(int i) { return (i - 1) / 2; }
int left(int i) { return (2 * i + 1); }
int right(int i) { return (2 * i + 2); }
int extractMin(); // extracts root (minimum) element
int getMin() { return harr[0]; }
// to replace root with new node x and heapify() new
// root
void replaceMin(int x)
{
harr[0] = x;
MinHeapify(0);
}
};
MinHeap::MinHeap(int a[], int size)
{
heap_size = size;
harr = a; // store address of array
}
void MinHeap::buildHeap()
{
int i = (heap_size - 1) / 2;
while (i >= 0) {
MinHeapify(i);
i--;
}
}
// Method to remove minimum element
// (or root) from min heap
int MinHeap::extractMin()
{
if (heap_size == 0)
return INT_MAX;
// Store the minimum vakue.
int root = harr[0];
// If there are more than 1 items,
// move the last item to
// root and call heapify.
if (heap_size > 1) {
harr[0] = harr[heap_size - 1];
MinHeapify(0);
}
heap_size--;
return root;
}
// A recursive method to heapify a subtree with root at
// given index This method assumes that the subtrees are
// already heapified
void MinHeap::MinHeapify(int i)
{
int l = left(i);
int r = right(i);
int smallest = i;
if (l < heap_size && harr[l] < harr[i])
smallest = l;
if (r < heap_size && harr[r] < harr[smallest])
smallest = r;
if (smallest != i) {
swap(&harr[i], &harr[smallest]);
MinHeapify(smallest);
}
}
// A utility function to swap two elements
void swap(int* x, int* y)
{
int temp = *x;
*x = *y;
*y = temp;
}
// Function to return k'th largest element from input stream
void kthLargest(int k)
{
// count is total no. of elements in stream seen so far
int count = 0, x; // x is for new element
// Create a min heap of size k
int* arr = new int[k];
MinHeap mh(arr, k);
while (1) {
// Take next element from stream
cout << "Enter next element of stream ";
cin >> x;
// Nothing much to do for first k-1 elements
if (count < k - 1) {
arr[count] = x;
count++;
}
else {
// If this is k'th element, then store it
// and build the heap created above
if (count == k - 1) {
arr[count] = x;
mh.buildHeap();
}
else {
// If next element is greater than
// k'th largest, then replace the root
if (x > mh.getMin())
mh.replaceMin(x); // replaceMin calls
// heapify()
}
// Root of heap is k'th largest element
cout << "K'th largest element is "
<< mh.getMin() << endl;
count++;
}
}
}
// Driver program to test above methods
int main()
{
int k = 3;
cout << "K is " << k << endl;
kthLargest(k);
return 0;
}
Coding Example #2: Write a function that finds the minimum length of an unsorted subarray
#include<bits/stdc++.h>
using namespace std;
void printUnsorted(int arr[], int n)
{
int s = 0, e = n-1, i, max, min;
// step 1(a) of above algo
for (s = 0; s < n-1; s++)
{
if (arr[s] > arr[s+1])
break;
}
if (s == n-1)
{
cout << "The complete array is sorted";
return;
}
// step 1(b) of above algo
for(e = n - 1; e > 0; e--)
{
if(arr[e] < arr[e-1])
break;
}
// step 2(a) of above algo
max = arr[s]; min = arr[s];
for(i = s + 1; i <= e; i++)
{
if(arr[i] > max)
max = arr[i];
if(arr[i] < min)
min = arr[i];
}
// step 2(b) of above algo
for( i = 0; i < s; i++)
{
if(arr[i] > min)
{
s = i;
break;
}
}
// step 2(c) of above algo
for( i = n -1; i >= e+1; i--)
{
if(arr[i] < max)
{
e = i;
break;
}
}
// step 3 of above algo
cout << "The unsorted subarray which"
<< " makes the given array" << endl
<< "sorted lies between the indees "
<< s << " and " << e;
return;
}
int main()
{
int arr[] = {10, 12, 20, 30, 25,
40, 32, 31, 35, 50, 60};
int arr_size = sizeof(arr)/sizeof(arr[0]);
printUnsorted(arr, arr_size);
getchar();
return 0;
}
Amazon Behavioral Questions
- Can you describe a time when you took the lead on a project?
- You witness a co-worker stealing. The value of the item is $2. What is your response?
- Describe a time when you had a set amount of time to complete a task.
- When you have the interests of large numbers of customers to think about, how do you prioritize their needs?
- Thinking about your current role, tell me about something that you have learned recently.
- Imagine a situation where separate managers have given you the same work to do, but that the priorities on each are different. What do you do?
- Tell me about a time you failed to meet a deadline. How did you cope with that?
- Have you ever faced a complex problem and managed to come up with a simple solution? Tell me about that.
- What is your proudest professional achievement?
- Why do you want to work for Amazon?
- Have you ever had to make a quick customer service decision without any help or guidance from a co-worker or manager? How did you arrive at a decision?
- Tell me about a time you have disagreed with your manager and how you handled it.
- How do you motivate others? Can you give me an example of a time you have motivated someone?
- You are asked by a supervisor to do something that is against policy and is unsafe. How would you handle that?
- Tell me about a time when you have had to work to earn someone’s trust.
- How do you deal with having to provide feedback to someone?
- Tell me about a time when you had to deal with ambiguity. How did you overcome the ambiguity to reach a positive outcome?
- Think about a time you received negative feedback. How did you deal with that?
- Can you describe a time when you took the lead on a project?
- Can you give me an example of a time when you exceeded expectations?
- Tell me about a time when you were working on a project, and you realized that you needed to make changes to what you were doing. How did you feel about the work you had already completed?
- Tell me about your most challenging customer. How did you resolve their issues and make them satisfied?
- Describe a time when you had to make a decision without having all the data or information you needed.
- Which Amazon leadership principle resonates with you most?
- How do you motivate co-workers to work together as a team?
How To Get A FAANG Job (The Easy Way)
Click below to claim your $400 discount on Tech Interview Pro today. Offer ends July 28, 2024.
CLAIM MY $400 SAVINGS
Offer ends July 28, 2024.Amazon Behavioral Answer Examples
Behavioral Example #1: Tell me about a time you have disagreed with your manager and how you handled it
To answer this question, talk about what led to the conflict between you and your boss and any necessary background information.
The biggest thing is to discuss why the disagreement came up: whether it’s related to lack of communication or a difference of opinion, provide the full details. When you paint the scene well, the interviewer can picture what happened and it sets you up for the rest of your answer.
Behavioral Example #2: Can you describe a time when you took the lead on a project?
To answer this question, choose an example where you delivered on time and within budget results. Talk about how you utilized both the people and non-people resources available to you to deliver the expected results.
If you haven't actually managed people or projects, substitute the word “led” for managed. In most cases, even individual contributors have led people and/or projects.
Behavioral Example #3: Which Amazon leadership principle resonates with you most?
To answer this question, you should pick something relevant for your job, and something that matches your personality.
For example, if you apply for a creative position with Amazon (something in software development, marketing, process engineering, etc), you can choose principle no. 3: Invent and simplify.
Why Tech Interview Pro?
So, some of these questions can be pretty easy to tackle.
But as I’ve mentioned in our Microsoft article, you can’t rely on getting only questions rated Easy to get through your interviews.
You’re just as likely to get every question to be rated Hard, and if you really want to have the best possible chance of success, it’d be better to study like if that were the case.
But how do you go about efficiently studying if you’re going to study that hard?
I recommend you use Tech Interview Pro, an interview prep program designed by a former Google and Facebook software engineer who has compressed the knowledge he gathered from conducting over 100 interviews during his time into a single course.
And here are a few reasons why I recommend it.
#1 A Comprehensive Approach
Tech Interview Pro takes a thorough approach to its teaching, taking both the technical and behavioral aspects into consideration in its 20+ hours of video lessons.
Here’s TIP’s course outline:
- Understanding The Interview Process
- Four Axes of the Interview
- Coding
- Data Structures & Algorithms
- Systems Design
- Communication
- Interviewing Masterclass
- Data Structures & Algorithms
- 100+ Coding Sessions
- The Mock Interview
- Systems Design
- Transitioning Careers
- Negotiating & Accepting Your Offer
As you can see, TIP has a balance of both the technical and the behavioral, which is definitely more coverage than most of the tech interview prep courses out there.
#2 Private Facebook Group
Another neat feature that Tech Interview Pro grants its students is access to a private Facebook group with 1,500+ former students of the course.
Here are some of their success stories:
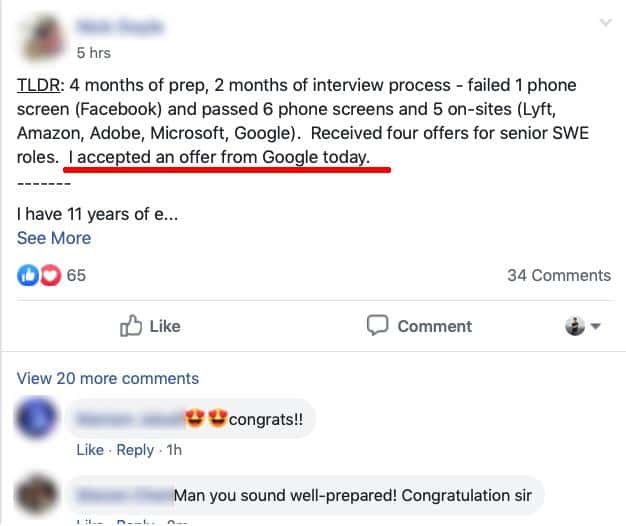
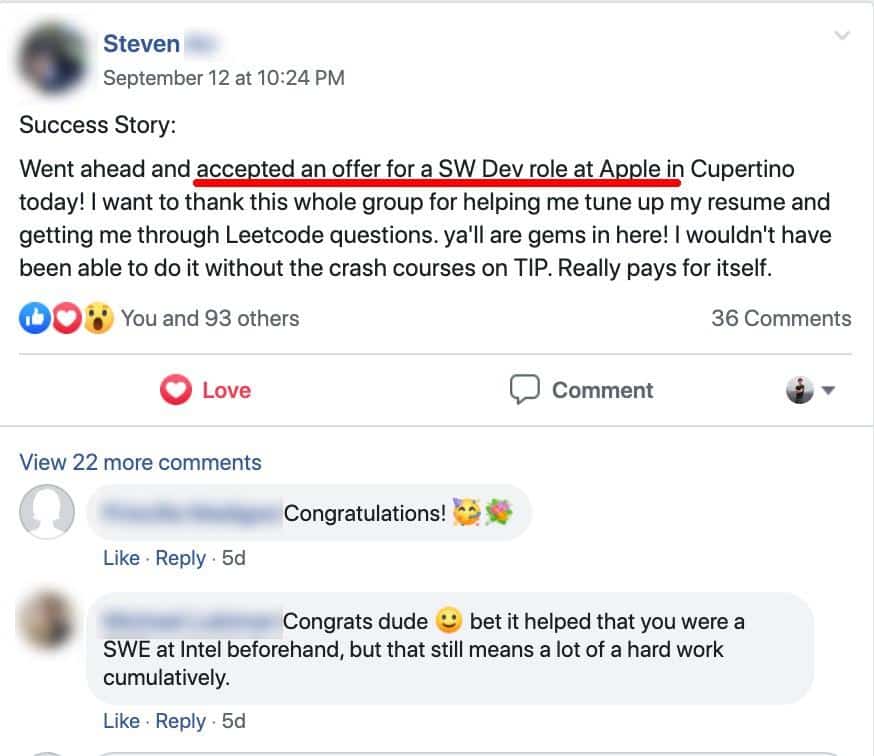
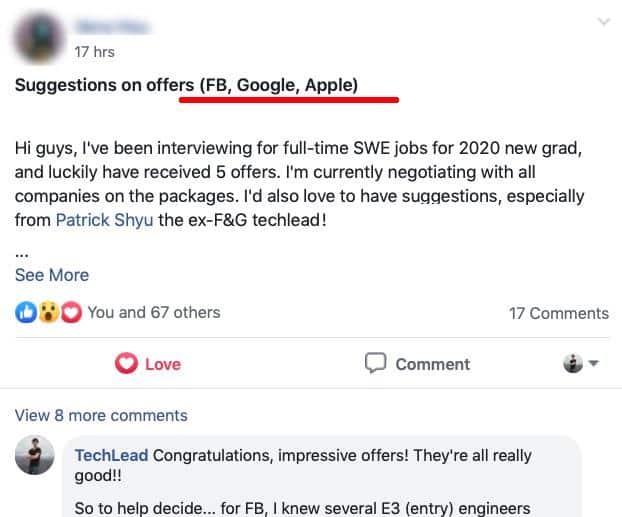
When you consider how many TIP students have landed jobs at FAANG companies thanks to the course, as well as how active and supportive the Facebook group is day to day, it’s really an invaluable resource to have.
#3 Created By An Industry Insider
Tech Interview Pro is run by an industry veteran who has reverse-engineered the hiring process to give you the best chance of success.
TechLead (Patrick Shyu) has grown his own websites to millions of users, spent his recent years working at Facebook and Google, and has conducted over 100 technical interviews for Google.
So if there’s anyone who can teach you how to ace your technical interviews, it’s this guy.
#4 Bi-Weekly Q&A Sessions
Every two weeks, TIP students can engage in Q&A sessions with the course’s founder, TechLead.
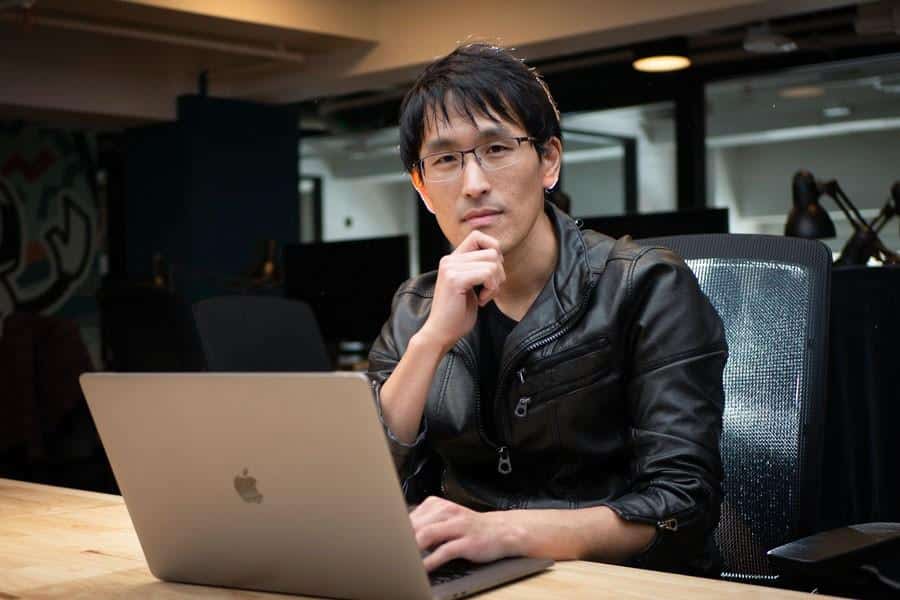
This is a huge advantage: after all, nothing’s stopping you from asking any of the questions featured on the list above, whether it’s a technical question or a behavioral one, and you can expect a thorough answer in return.
Another thing to keep in mind is that every Q&A session is recorded and ready to be accessed at any time, so there’s nothing stopping you from checking previous Q&A sessions too.
#5 Resume Reviews
Your resume will be the first thing a recruiter will see coming from your end, and making it look pretty won’t be enough: you need to make sure it knocks them out when they see it so that you can have a headstart into the interviewing process.
Considering this, TechLead will also personally review your resume and help you tailor it for the position and the company you seek to apply for.
#6 Lifetime Access
Lastly, TIP grants lifetime access to the entire course and private Facebook group, which means that you’ll be able to see new video lessons as they’re added, grow alongside the Facebook group, watch all of future Q&A sessions, and everything else.
TIP’s got your back, whether you want to land a job now or in the future.
In Summary
Getting into Amazon as a software engineer is no walk in the park, and most of their technical and behavioral questions can be pretty tricky to handle. As long as you prepare for these questions in advance, though, you stand a good chance of getting the job.
I strongly recommend Tech Interview Pro if you're looking for a proven program that's landed thousands of engineers six-figure jobs at Facebook, Google, Amazon, and Apple, I strongly recommend Tech Interview Pro. With a money-back guarantee and thousands of student success stories, I can't recommend Tech Interview Pro highly enough.